How to Read Fhir Bundle File Python
Overview
When you're working with Python, you don't need to import a library in order to read and write to a file. It's handled natively in the language, admitting in a unique manner. Beneath, nosotros outline the simple steps to read and write to a file in Python.
The first thing you'll need to exercise is use the congenital-in python open file function to get a file object .
The open function opens a file. Information technology'due south unproblematic. This is the first step in reading and writing files in python.
When you use the open part, it returns something chosen a file object . File objects contain methods and attributes that tin be used to collect information about the file you lot opened. They can also be used to manipulate said file.
For example, the style attribute of a file object tells you which mode a file was opened in. And the name attribute tells you the name of the file.
You must understand that a file and file object are ii wholly split up – yet related – things.
File Types
What you may know as a file is slightly dissimilar in Python.
In Windows, for instance, a file tin be any item manipulated, edited or created past the user/Os. That means files tin exist images, text documents, executables, and excel file and much more. Most files are organized by keeping them in individual folders.
A file In Python is categorized as either text or binary, and the difference between the two file types is of import.
Text files are structured as a sequence of lines, where each line includes a sequence of characters. This is what yous know as code or syntax.
Each line is terminated with a special grapheme, chosen the EOL or End of Line character. There are several types, but the most common is the comma {,} or newline character. It ends the current line and tells the interpreter a new ane has begun.
A backslash character can as well be used, and it tells the interpreter that the next character – following the slash – should be treated as a new line. This grapheme is useful when you don't desire to commencement a new line in the text itself just in the code.
A binary file is any type of file that is not a text file. Because of their nature, binary files tin can only be processed by an application that know or sympathize the file's construction. In other words, they must be applications that can read and interpret binary.
Reading Files in Python
In Python, files are read using the open() method. This is one of Python's congenital-in methods, fabricated for opening files.
The open() function takes two arguments: a filename and a file opening fashion. The filename points to the path of the file on your computer, while the file opening mode is used to tell the open() role how we programme to interact with the file.
Past default, the file opening manner is set to read-only, meaning we'll only have permission to open and examine the contents of the file.
On my computer is a folder called PythonForBeginners. In that binder are iii files. One is a text file named emily_dickinson.txt, and the other ii are python files: read.py and write.py.
The text file contains the following poem, written by poet Emily Dickinson. Perhaps we are working on a poetry programme and have our poems stored as files on the calculator.
Success is counted sweetest
By those who ne'er succeed.
To embrace a nectar
Requires sorest demand.
Non one of all the Imperial Host
Who took the Flag today
Can tell the definition
So clear of Victory
As he defeated, dying
On whose forbidden ear
The distant strains of triumph
Flare-up agonized and clear.
Before we can do anything with the contents of the verse form file, nosotros'll need to tell Python to open information technology. The file read.py, contains all the python code necessary to read the poem.
Any text editor tin be used to write the code. I'm using the Atom lawmaking editor, which is my editor of choice for working in python.
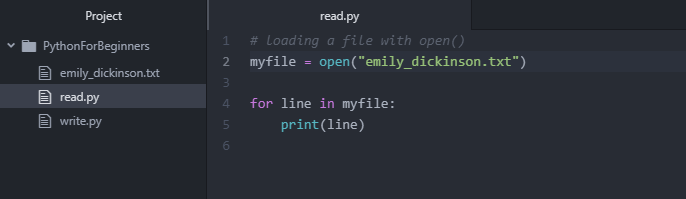
# read.py # loading a file with open() myfile = open("emily_dickinson.txt") # reading each line of the file and printing to the panel for line in myfile: print(line)
I've used Python comments to explicate each step in the code. Follow this link to learn more well-nigh what a Python comment is.
The example in a higher place illustrates how using a simple loop in Python can read the contents of a file.
When it comes to reading files, Python takes care of the heaving lifting backside the scenes. Run the script by navigating to the file using the Control Prompt — or Terminal — and typing 'python' followed by the name of the file.
Windows Users: Before you can use the python keyword in your Command Prompt, you'll need to set up the environment variables. This should have happened automatically when you installed Python, but in case it didn't, yous may demand to practice it manually.
>python read.py
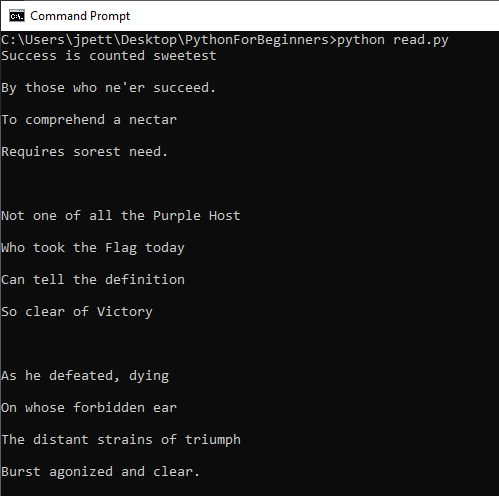
Information provided by the open up() method is usually stored in a new variable. In this instance, the contents of the verse form are stored in the variable "myfile."
Once the file is created, we can use a for loop to read every line in the file and impress its contents to the command line.
This is a very elementary instance of how to open a file in Python, just educatee'southward should be enlightened that the open() method is quite powerful. For some projects information technology will be the only affair needed to read and write files with Python.
Writing Files in Python
Before we can write to a file in Python, it must first be opened in a different file opening way. Nosotros tin do this by supplying the open() method with a special argument.
In Python, write to file using the open() method. You'll demand to laissez passer both a filename and a special character that tells Python we intend to write to the file.
Add together the following lawmaking to write.py. We'll tell Python to look for a file named "sample.txt" and overwrite its contents with a new message.
# open the file in write style myfile = open("sample.txt",'westward') myfile.write("Hello from Python!")
Passing 'westward' to the open() method tells Python to open the file in write style. In this style, whatsoever data already in the file is lost when the new information is written.
If the file doesn't exist, Python will create a new file. In this case, a new file named "sample.txt" will be created when the plan runs.
Run the program using the Command Prompt:
>python write.py
Python tin can as well write multiple lines to a file. The easiest manner to exercise this is with the writelines() method.
# open the file in write style myfile = open up("sample.txt",'w') myfile.writelines("Hi World!","We're learning Python!") # shut the file myfile.close()
We can besides write multiple lines to a file using special characters:
# open up the file in write mode myfile = open up("poem.txt", 'westward') line1 = "Roses are blood-red.\northward" line2 = "Violets are blue.\due north" line3 = "Python is groovy.\n" line4 = "And so are you.\n" myfile.write(line1 + line2 + line3 + line4)
Using string concatenation makes it possible for Python to salve text data in a variety of ways.
However, if nosotros wanted to avert overwriting the data in a file, and instead suspend information technology or change it, we'd take to open up the file using another file opening style.
File Opening Modes
By default, Python will open the file in read-just mode. If we desire to do anything other than just read a file, we'll need to manually tell Python what nosotros intend to do with information technology.
- 'r' – Read Mode: This is the default mode for open() . The file is opened and a arrow is positioned at the beginning of the file's content.
- 'w' – Write Manner: Using this mode volition overwrite whatsoever existing content in a file. If the given file does not be, a new 1 will be created.
- 'r+' – Read/Write Fashion: Use this mode if you demand to simultaneously read and write to a file.
- 'a' – Append Mode: With this mode the user can append the data without overwriting any already existing data in the file.
- 'a+' – Append and Read Mode: In this mode you tin read and append the data without overwriting the original file.
- 'x' – Sectional Creating Mode: This fashion is for the sole purpose of creating new files. Use this mode if you lot know the file to be written doesn't exist beforehand.
Note: These examples presume the user is working with text file types. If the intention is to read or write to a binary file type, an additional argument must exist passed to the open() method: the 'b' character.
# binary files need a special argument: 'b' binary_file = open("song_data.mp3",'rb') song_data = binary_file.read() # shut the file binary_file.close()
Closing Files with Python
After opening a file in Python, information technology's important to shut it after you're done with it. Closing a file ensures that the plan tin no longer access its contents.
Close a file with the shut() method.
# open a file myfile = open("poem.txt") # an array to shop the contents of the file lines = [] For line in myfile: lines.append(line) # close the file myfile.shut() For line in liens: print(line)
Opening Other File Types
The open() method can read and write many unlike file types. We've seen how to open up binary files and text files. Python can also open images, allowing you lot to view and edit their pixel information.
Earlier Python can open an image file, the Pillow library (Python Imaging Library) must exist installed. It'south easiest to install this module using pip.
pip install Pillow
With Pillow installed, Python can open paradigm files and read their contents.
From PIL import Image # tell Pillow to open the image file img = Image.open("your_image_file.jpg") img.show() img.close()
The Pillow library includes powerful tools for editing images. This has made information technology ane of the most popular Python libraries.
With Argument
You can also work with file objects using the with statement. It is designed to provide much cleaner syntax and exceptions handling when you are working with code. That explains why it's proficient practice to utilise the with argument where applicative.
One bonus of using this method is that whatever files opened will exist closed automatically after you are done. This leaves less to worry about during cleanup.
To apply the with statement to open a file:
with open("filename") as file:
Now that you understand how to call this argument, permit's take a look at a few examples.
with open("poem.txt") as file: data = file.read() practise something with information
Y'all can also call upon other methods while using this argument. For instance, you can do something similar loop over a file object:
due westith open("poem.txt") every bit f: for line in f: print line,
You'll also find that in the above example nosotros didn't use the " file.close() " method because the with statement will automatically call that for us upon execution. It really makes things a lot easier, doesn't it?
Splitting Lines in a Text File
As a final case, permit's explore a unique function that allows you to split the lines taken from a text file. What this is designed to do, is dissever the cord contained in variable information whenever the interpreter encounters a infinite grapheme.
But merely because we are going to employ it to split up lines subsequently a space character, doesn't mean that's the just way. Y'all can actually split your text using any character you lot wish – such as a colon, for instance.
The code to do this (too using a with statement) is:
with open( " hello.text ", "r") as f: data = f.readlines () for line in data: words = line.separate () print words
If yous wanted to use a colon instead of a space to split your text, you would simply change line.divide() to line.carve up(":").
The output for this volition be:
["hello", "world", "how", "are", "y'all", "today?"] ["today", "is", "Saturday"]
The reason the words are presented in this fashion is because they are stored – and returned – equally an array. Be sure to remember this when working with the split office.
Conclusion
Reading and writing files in Python involves an understanding of the open() method. By taking advantage of this method's versatility, it's possible to read, write, and create files in Python.
Files Python can either be text files or binary files. It'southward also possible to open and edit prototype data using the Pillow module.
Once a file'due south data is loaded in Python, in that location's almost no end to what can be done with it. Programmers ofttimes work with a large number of files, using programs to generate them automatically.
Every bit with any lesson, there is only so much that can be covered in the space provided. Hopefully yous've learned enough to go started reading and writing files in Python.
More than Reading
Official Python Documentation – Reading and Writing Files
Python File Handling Cheat Canvas
Recommended Python Training
Grade: Python 3 For Beginners
Over fifteen hours of video content with guided didactics for beginners. Learn how to create real world applications and master the basics.
Source: https://www.pythonforbeginners.com/files/reading-and-writing-files-in-python
0 Response to "How to Read Fhir Bundle File Python"
Postar um comentário